Mastering Asynchronous Programming in Unity with UniTask and DOTween
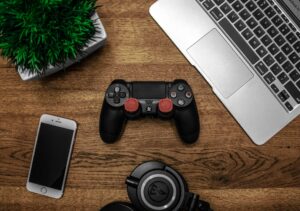
In the ever-evolving landscape of game development, efficient asynchronous programming is crucial for creating smooth and responsive game experiences. Unity developers have traditionally relied on coroutines for async operations, but there’s a more powerful and efficient alternative: UniTask. When combined with the popular tweening library DOTween, UniTask enables developers to create complex async workflows with ease.
— Ads —
Why UniTask?
UniTask is a lightweight, allocation-free async/await library designed specifically for Unity. Unlike traditional coroutines, UniTask leverages C#’s async/await pattern, making your code more readable, maintainable, and performant. Here are some key benefits of using UniTask:
- Zero Allocation: UniTask aggressively caches async promise objects, resulting in zero memory allocation after the initial cache setup. This makes it ideal for performance-critical scenarios like game loops.
- Better Error Handling: UniTask provides superior error handling compared to coroutines, with try/catch support and better exception management.
- Seamless Integration: UniTask integrates smoothly with popular Unity plugins like DOTween, maintaining its zero-allocation benefits while providing powerful async capabilities.
- Familiar Async/Await Syntax: UniTask uses the familiar async/await pattern, making it easier for developers comfortable with C#’s async programming model.
Getting Started with UniTask
Installation
To install UniTask, you can use the Unity Package Manager with the following Git URL:
https://github.com/Cysharp/UniTask.git?path=src/UniTask/Assets/Plugins/UniTask
For more details, check out the UniTask GitHub repository.
Basic Usage
Here’s a simple example of using UniTask to delay an operation by 10 seconds:
await UniTask.Delay(TimeSpan.FromSeconds(10), ignoreTimeScale: false);
In this example, ignoreTimeScale: false
ensures that the delay respects Unity’s time scale, pausing when the game is paused.
UniTask and DOTween: A Powerful Combination
DOTween is a popular tweening library for Unity that integrates seamlessly with UniTask. To use UniTask with DOTween, first install DOTween, then install UniTask. If you install UniTask first, you’ll need to add UNITASK_DOTWEEN_SUPPORT
under Scripting Define Symbols in your Project Settings.
Installation Order
- Install DOTween.
- Install UniTask via the Unity Package Manager using the Git URL provided above.
Using UniTask with DOTween
Here’s an example of using UniTask with DOTween to move a GameObject over 2 seconds:
await transform.DOMove(new Vector3(10, 0, 0), 2f).ToUniTask();
In this example, ToUniTask()
converts the DOTween sequence to a UniTask, allowing you to await its completion.
Advanced UniTask Usage
Waiting for Multiple Tasks
UniTask makes it easy to wait for multiple tasks to complete using UniTask.WhenAll
. Here’s an example of fetching text from multiple web requests concurrently:
var task1 = GetTextAsync(UnityWebRequest.Get("http://google.com"));
var task2 = GetTextAsync(UnityWebRequest.Get("http://bing.com"));
var task3 = GetTextAsync(UnityWebRequest.Get("http://yahoo.com"));
// Concurrent async-wait and get results easily by tuple syntax
var (google, bing, yahoo) = await UniTask.WhenAll(task1, task2, task3);
// Shorthand of WhenAll, tuple can await directly
var (google2, bing2, yahoo2) = await (task1, task2, task3);
Timing Control
UniTask provides precise control over timing, allowing you to wait for specific Unity update cycles, create frame-based delays, and perform time-scaled operations. Here are some examples:
// Wait for the next frame
await UniTask.NextFrame();
// Wait for the next end of frame
await UniTask.EndOfFrame();
// Wait for a specific number of frames
await UniTask.DelayFrame(10);
// Time-scaled delay
await UniTask.Delay(TimeSpan.FromSeconds(10), ignoreTimeScale: true);
Error Handling
UniTask’s superior error handling makes it easier to manage exceptions in your async workflows. Here’s an example of using try/catch with UniTask:
try
{
await SomeAsyncOperation();
}
catch (Exception ex)
{
Debug.LogError($"Operation failed: {ex.Message}");
}
Cancellation
Proper task cancellation is crucial for managing resources and preventing memory leaks. Here’s an example of canceling a UniTask:
var cancellationTokenSource = new CancellationTokenSource();
var task = SomeAsyncOperation(cancellationTokenSource.Token);
// Cancel the task
cancellationTokenSource.Cancel();
Migrating from Coroutines
Migrating existing coroutine code to UniTask can significantly improve your code’s readability and performance. Here are some tips for migrating:
- Replace
yield return
withawait
: Most coroutineyield return
statements can be replaced withawait
. - Use
UniTask.Delay
instead ofWaitForSeconds
: Replaceyield return new WaitForSeconds(...)
withawait UniTask.Delay(...)
. - Convert Anonymous IEnumerator Methods: Convert anonymous IEnumerator methods to async methods returning
UniTask
.
Performance Optimization Tips
To maximize UniTask’s performance benefits, follow these best practices:
- Avoid State Machines: UniTask’s zero-allocation design makes it ideal for replacing complex state machines with simple async methods.
- Cache Reusable Tasks: Cache and reuse UniTask objects whenever possible to minimize memory allocation.
- Use ConfigPool: For performance-critical scenarios, use
UniTask.ConfigPool
to configure the task pool size and optimize memory usage.
Conclusion
UniTask is a powerful and efficient alternative to traditional coroutines for asynchronous programming in Unity. When combined with DOTween, UniTask enables developers to create complex async workflows with ease, improving code readability, maintainability, and performance.
To learn more about using UniTask and DOTween in Unity, check out this informative video tutorial.
Happy coding, and until next time, keep your async workflows smooth and efficient with UniTask and DOTween!